Express Async Errors: A Complete Guide to Error Handling
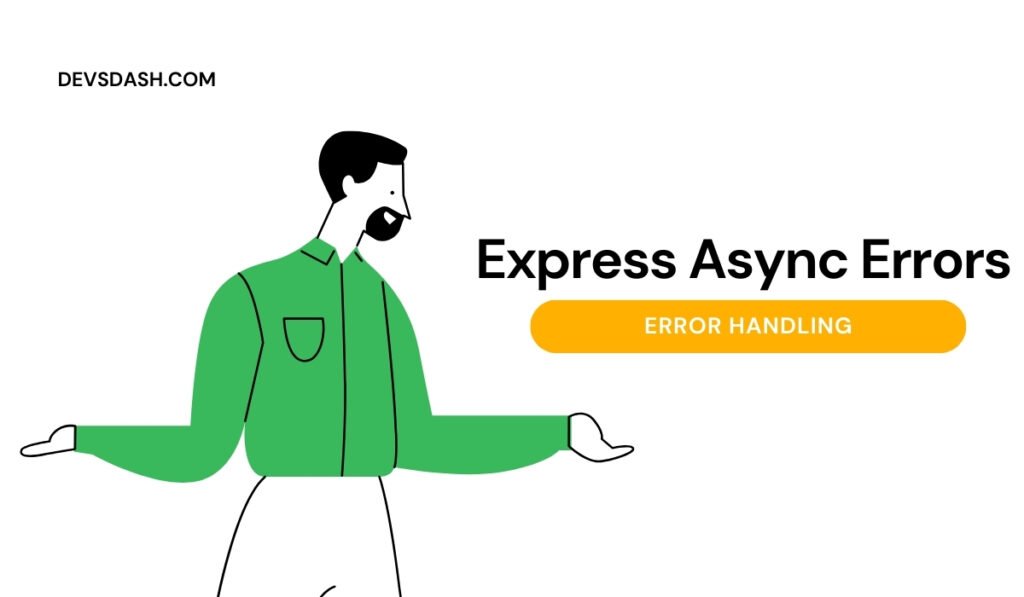
Express Async Errors
In this tutorial, I’m going to show how to use the Express-Async-Errors package in your applications and how to create error middleware to catch errors.
Sound good? Let’s get started.
What is Express-Async-Errors
Express-Async-Errors library is an amazing way to catch errors at runtime without using try/catch blocks in your async functions.
Install Express-Async-Errors library
Open the terminal and navigate to your project directory, then run the following command:
npm install express-async-errors --save
Express-Async-Errors usage example
If you import it now into your project, nothing will happen because we need to create an error middleware first.
Create the error middleware
When an error occurs it will be sent to this middleware so you can do amazing things like:
- Log the error. You can save the error in a log file or store it in your database so you can look at it later and fix the issue.
- Send a response back to the client. When an error occurs the request that’s sent by the client will be left hanging with no response so the error middleware will allow you to send a response back like a 500 server error.
Now let’s implement the error middleware.
Create an error.js file in your project with the following code:
module.exports = function (err, req, res, next) {
//respond with a 500 server error
res.status(500).send("This is a server error by express-async-erros");
};
Express-Async-Errors setup
Now we are ready to use the express-async-errors library in our project.
- Import the library. You have to import the library before creating any routes.
- Import the error middleware. The library will pass the request to the error middleware if any error occurs.
- Create your routes. All the async functions inside these routes will be patched with try/catch under the hood.
- Use The error middleware. Handle errors that occur inside your Async functions
require("express-async-errors");
const express = require("express");
const error = require("./error.js");
const app = express();
app.get("/", async (req, res) => {
throw new Error("error");
});
app.use(error);
app.listen(3000);