Twitch API Javascript example with source code
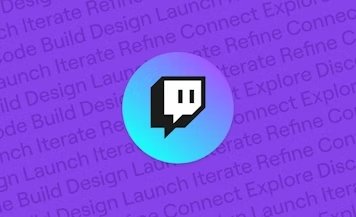
Twitch API Javascript
After this tutorial, you will be able to read different types of data from Twitch using their API in Javascript.
Sound good? Let’s get started.
Setting up your Twitch application
Just like every major company API you need to create an application for each of your projects so they can authenticate you and track your usage.
Step #1. Sign In / Sign Up for a Twitch account.
Go to Twitch Developers and click on the Login in with Twitch button on the top right corner.
Step #2. Register your application.
After signing in you should be directed to the Console Page. Once you’re there click on the Register your Application button.
Now, fill yin our application information (Name, OAuth Redirect URLs, Category).
Because we will use the Twitch API on our local machine here’s how we would fill this information.
After you click Create, Twitch might ask you to activate two-factor authentication for your account.
If this happens to you, Go to your account settings -> security and privacy -> enable two-factor authentication.
Getting your Twitch application credentials
To connect to Twitch API you need two main things:
- Client ID: You can get your Client ID from the Twitch developers application tab then click manage on your application.
- Client Secret: Click on the New Secret button to generate one.
Now that you have both Client ID and Client Secret, You can get your Authorization Object.
let clinetId = "CLIENT_ID_HERE";
let clinetSecret = "CLINET_SECRET_HERE";
function getTwitchAuthorization() {
let url = `https://id.twitch.tv/oauth2/token?client_id=${clinetId}&client;_secret=${clinetSecret}&grant;_type=client_credentials`;
fetch(url, {
method: "POST",
})
.then((res) => res.json())
.then((data) => handleAuthorization(data));
}
function handleAuthorization(data) {
let { access_token, expires_in, token_type } = data;
document.write(`${token_type} ${access_token}`);
}
getTwitchAuthorization();
When you place your Client ID and Client Secret in the code above, run the code and you will see similar result in your web browser:
Twitch API example in Javascript
Now that you have everything you need to connect, You can choose any endpoint to connect to.
There are a lot of different endpoints you can connect to, for example (Get Clips, Get Top Games, Get Streams, etc).
However, for this example, we’re going to use the Get Streams endpoint.
You can view all different endpoints from the Twitch API Reference.
The code below will request the Authorization Object then request all live streams and print the data on the browser as JSON.
let clinetId = "CLIENT_ID_HERE";
let clinetSecret = "CLINET_SECRET_HERE";
function getTwitchAuthorization() {
let url = `https://id.twitch.tv/oauth2/token?client_id=${clinetId}&client;_secret=${clinetSecret}&grant;_type=client_credentials`;
return fetch(url, {
method: "POST",
})
.then((res) => res.json())
.then((data) => {
return data;
});
}
async function getStreams() {
const endpoint = "https://api.twitch.tv/helix/streams";
let authorizationObject = await getTwitchAuthorization();
let { access_token, expires_in, token_type } = authorizationObject;
//token_type first letter must be uppercase
token_type =
token_type.substring(0, 1).toUpperCase() +
token_type.substring(1, token_type.length);
let authorization = `${token_type} ${access_token}`;
let headers = {
authorization,
"Client-Id": clinetId,
};
fetch(endpoint, {
headers,
})
.then((res) => res.json())
.then((data) => renderStreams(data));
}
function renderStreams(data) {
document.body.innerHTML = `
${JSON.stringify(data)}
`; } getStreams();
When you run the code you will see a similar result in your web browser: (Yours will appear as a big chunk of text)
And this is it, now you have all the live streams on Twitch in a JSON object and ready to be used.
As a bonus let’s create a HTML page to present these data.
The code viewer below contains the final code of -> index.html, style.css, and twitch.js.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Twitch Live Streams Example - DevsDash.com</title>
<link rel="preconnect" href="https://fonts.googleapis.com" />
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin />
<link
href="https://fonts.googleapis.com/css2?family=Poppins:wght@400;600;800&display=swap"
rel="stylesheet"
/>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h1>Twitch Live Streams</h1>
<div class="streams"></div>
</body>
<script src="twitch.js"></script>
</html>
When you run the code you will see a similar result in your web browser: